LCD displej EA-DOGM 163
Zo stránky SensorWiki
LCD Modul s displejom EA-DOGM-163
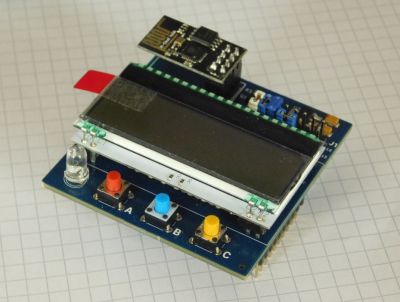
Toto je návod na cvičenie s doskou Acrob a rozširujúcim modulom EA-DOGM163. Ak chcete pracovať s bežným LCD displejom s paralelným pripojením, pozrite sa na tento návod pre LCD displej s radičom HD44780.
Vlastnosti:
- 3x16 LCD Displej s podsvietením
- 3 Tlačítka, 1 LED
- Príprava na ESP-01 WiFi modul
- Konfiguračné prepojky
Technické parametre:
- Napájanie: 5 V @ 15 mA
- Pripojenie: SPI interface
- Radič displeja je Hitachi HD44780 compatible
- Rozmery: 60 x 50 x 20 mm
- Pracovná teplota: 0 až +70 °C
Na rozširujúcej doske s displejom máte k dispozícii 3-riadkový displej EA-DOGM163 s podsvietením a okrem toho ešte jednu LED diódu navyše
a tri tlačidlá, ktoré môžete použiť na svoje vlastné účely. Pred pripojením dosky hrebienkovým konektorom k základnej doske Acrob odpojte
napätie a skontrolujte orientáciu a polohu pinov.
Rozmiestnenie prepojok na konektore J1 'nemeňte', môžete však skontrolovať ich správnu polohu podľa obr. 1.
Po pripojení modulu k doske Acrob sú obsadené nasledovné vstupy a výstupy mikroprocesora, ktoré už ďalej nemôžete používať na svoje vlastné
účely.
AVR Pin Arduino Význam signálu PORTD.2 D2 LCD: RS (SPI zbernica, Register Select) PORTD.4 D4 LCD: /CS (displej, Chip Select) PORTD.6 D6 LCD: Backlight - podsvietenie displeja On/Off alebo plynule PWM PORTD.7 D7 SW_B Prostredné tlačidlo PORTB.0 D8 SW_C Pravé tlačidlo PORTB.3 D11 LCD: SI PORTB.5 D13 LCD: CLK PORTC.2 A2 LED na module vľavo PORTC.3 A3 SW_A Ľavé tlačidlo
Piny, ktoré využíva LCD displej pripojený cez zbernicu SPI, nemusíte konfigurovať, všetko zabezpečí knižnica lcd. Súbory lcd.c a lcd.h podľa možnosti nemeňte a nezabudnite na ich správne vloženie do projektu. Pre správnu funkciu potrebujete svoje vlastné knižnice uart.c a uart.h (alebo vypustite príslušné časti kódu z ukážky).
/*
* LcdDemo.c Ver 0.3 (15. 4. 2025 19:53:07)
* Ver 0.2 (10. 4. 2025 07:26:17)
* Ver 0.1 (10. 3. 2024 09:47:21)
*
* Minimal working example for the FEI STU
* Function:
* a) setup serial interface and prepare stdio.h
* b) setup LCD display an show some text on it
* c) student functions are not used in demo
*
* Necessary definitions set in Project -> Properties
* ATmega328P
* F_CPU=16000000UL
* BAUDRATE=9600
*
*/
#include <avr/io.h>
#include "uart.h"
#include "lcd.h"
#define LED1 PB5 // internal Acrob LED
#define LED2 PC2 // LCD board external LED
#define SW_A PC3 // Tlacitko vlavo - vsetky tri maju pullUp
#define SW_B PD7 // Tlacitko v strede
#define SW_C PB0 // Tlacitko vpravo
#include <stdio.h>
FILE mystdout = FDEV_SETUP_STREAM(uart_putc, NULL, _FDEV_SETUP_WRITE);
int main(void)
{
DDRB |= (1<<LED1); // PORTB.5 kde je LED ma byt OUTPUT
DDRC |= (1<<LED2); // Doplnujuca LED na displeji, Output
uart_init();
stdout = &mystdout; // printf() works from now
printf("Hello, world! \n"); // output to Serial terminal
lcd_init(); // inicializacia displeja
lcd_bklt(1); // zapni podsvietenie displeja
lcd_putc(0x2A); // 0x2A hex = 52 dec = '*'
lcd_putc(' '); // znak medzera
for (int i=0; i<26; i++) lcd_putc('A'+i);
lcd_putc(' '); // znak medzera
/* tato cast kodu zatial nefunguje, vasa uloha je
doprogramovat prislusne funkcie tak, aby to islo */
lcd_setCursor(3,3); // nastav kurzor na 3. riadok, 3. stlpec
lcd_puts("Hello, world!"); // a vypis tam tento text
while(1)
{
if (bit_is_clear(PINC, SW_A)) // Test tlacitka
{
lcd_putc('*'); // ak je stlacene, napis *
_delay_ms(1000); // a pockaj chvilu
}
}
return(0);
}
/*
* lcd.h Library for MISA @ FEI STU
*
* Created: 15. 4. 2025 20:48:48 - added char definition support, removed ext. dependencies
* Author: Richard Balogh based on gitHub code for EA*DOGM163
*/
#ifndef LCD_H_
#define LCD_H_
#include <stdlib.h>
#include <util/delay.h>
#include <avr/interrupt.h>
#include <avr/pgmspace.h>
#define CHARACTER_BUFFER_BASE_ADDRESS 0x80
#define CHARACTERS_PER_ROW 16
#define DISPLAY_RS_PORT PORTD
#define DISPLAY_RS_DDR DDRD
#define DISPLAY_RS_PIN 2
#define DISPLAY_CSB_PORT PORTD
#define DISPLAY_CSB_DDR DDRD
#define DISPLAY_CSB_PIN 4
#define DISPLAY_BKLT_PORT PORTD
#define DISPLAY_BKLT_DDR DDRD
#define DISPLAY_BKLT_PIN 6
#define DISPLAY_RS_low (DISPLAY_RS_PORT &= ~(1<<DISPLAY_RS_PIN))
#define DISPLAY_RS_high (DISPLAY_RS_PORT |= (1<<DISPLAY_RS_PIN))
#define DISPLAY_CSB_low (DISPLAY_CSB_PORT &= ~(1<<DISPLAY_CSB_PIN))
#define DISPLAY_CSB_high (DISPLAY_CSB_PORT |= (1<<DISPLAY_CSB_PIN))
#define DISPLAY_BKLT_low (DISPLAY_BKLT_PORT &= ~(1<<DISPLAY_BKLT_PIN))
#define DISPLAY_BKLT_high (DISPLAY_BKLT_PORT |= (1<<DISPLAY_BKLT_PIN))
//instructions (see the ST7036 instruction set for further information)
#define INSTRUCTION_CLEAR_DISPLAY 0b00000001
#define INSTRUCTION_FUNCTION_SET_INIT_0 0b00110011
#define INSTRUCTION_FUNCTION_SET_INIT_1 0b00110010
#define INSTRUCTION_FUNCTION_SET_INIT_2 0b00101001
#define INSTRUCTION_INSTRUCTION_SET_0 0b00101000
#define INSTRUCTION_INSTRUCTION_SET_1 0b00101001
#define INSTRUCTION_BIAS_SET 0b00010101
#define INSTRUCTION_POWER_CONTROL 0b01010011
#define INSTRUCTION_FOLLOWER_CONTROL 0b01101100
#define INSTRUCTION_CONTRAST_SET 0b01111111
#define INSTRUCTION_DISPLAY_ON 0b00001100
#define INSTRUCTION_ENTRY_MODE 0b00000110
void lcd_init(void);
void lcd_write( char data );
void lcd_data( char data );
void lcd_command( char instruction );
void lcd_putc( char znak );
void lcd_puts( char *string );
void lcd_clear( void );
void lcd_clearline( unsigned char zeile );
void lcd_setCursor(char row, char col);
void lcd_bklt( char OnOff);
void def_znak(unsigned char *ZnakArray, unsigned char Address);
#endif /* LCD_H_ */
#include "lcd.h"
/* Primitivne funkcie, nepredpoklada sa ich vyuzitie uzivatelom */
/* Funkcia zapise jeden bajt po SPI zbernici do zariadenia */
void lcd_write( char data )
{
signed char index = 8;
char c_data;
DISPLAY_CSB_PORT &= ~(1<<DISPLAY_CSB_PIN); // Chip-Select do log.0
c_data = data;
do
{
_delay_us(6);
if ( c_data & 0x80 ) // najyssi bit zamaskujeme
PORTB |= (1<<3); // a posleme ho na zbernicu
else
PORTB &= ~(1<<3); // cez vodic MOSI (alebo len SI)
_delay_us(5); // a vygenerujeme jeden hodinovy pulz
PORTB &= ~(1<<5); // na vyvod CLK
_delay_us(6);
PORTB |= (1<<5);
c_data = c_data << 1; // na najvyssie miesto posunieme dalsi bit
index--;
} while (index > 0); // a toto spravime dokola 8x
// a napokon zdvihneme /CS do log.1
_delay_ms( 2 );
DISPLAY_CSB_PORT |= (1<<DISPLAY_CSB_PIN);
}
/* Funkcia zapise jeden byte do riadiaceho (Control) registra */
void lcd_command( char instruction )
{
DISPLAY_RS_PORT &= ~(1<<DISPLAY_RS_PIN);
_delay_us( 1 );
lcd_write( instruction );
}
/* Funkcia zapise jeden byte do datoveho (Data) registra */
void lcd_data( char data )
{
DISPLAY_RS_PORT |= 1<<DISPLAY_RS_PIN;
_delay_us( 7 );
lcd_write( data );
}
/* Uzivatelske funkcie, ktore ma pouzivat uzivatel */
/* Inicializacia displeja podla datasheetu pre radic ST7036 */
void lcd_init(void)
{
DDRB |= (1<<PB3) | (1<<PB5); // MOSI + SCK as OUTPUTs
DISPLAY_RS_DDR |= 1<<DISPLAY_RS_PIN; // All signals as OUTPUT
DISPLAY_CSB_DDR |= 1<<DISPLAY_CSB_PIN;
DISPLAY_BKLT_DDR |= 1<<DISPLAY_BKLT_PIN;
PORTB |= (1<<PB5); // set_bit( ST7036_CLK );
DISPLAY_CSB_PORT |= (1<<DISPLAY_CSB_PIN); // set_bit( ST7036_CSB );
DISPLAY_RS_PORT &= ~(1<<DISPLAY_RS_PIN); // set_bit( ST7036_CSB );
_delay_ms(50); // pockame viac ako 40ms na ustalenie napajacieho napatia
lcd_command( 0x39 ); // Function set; 8-bit Datenlänge, 2 Zeilen, Instruction table 1
_delay_us(50); // mehr als 26,3µs warten
lcd_command( 0x1d ); // Bias Set; BS 1/5; 3 zeiliges Display /1d
_delay_us(50); // mehr als 26,3µs warten
lcd_command( 0x50 ); // Booster aus; Kontrast C5, C4 setzen /50
_delay_us(50); // mehr als 26,3µs warten
lcd_command( 0x6c ); // Spannungsfolger und Verstärkung setzen /6c
_delay_ms( 500 ); // mehr als 200ms warten !!!
lcd_command( 0x7c ); // Kontrast C3, C2, C1 setzen /7c
_delay_us(50); // mehr als 26,3µs warten
lcd_command( 0x38 ); // Display EIN, Cursor EIN, Cursor BLINKEN /0f
_delay_us(50); // mehr als 26,3µs warten
lcd_command( 0x0f ); // Display EIN, Cursor EIN, Cursor BLINKEN /0f
_delay_us(50); // mehr als 26,3µs warten
lcd_command( 0x01 ); // Display löschen, Cursor Home
_delay_ms(400); //
lcd_command( 0x06 ); // Cursor Auto-Increment
_delay_us(50); // mehr als 26,3µs warten
}
/* Funkcia zobrazi na pozicii kurzoru jeden znak */
/* Alias funkcia z dovodov kompatibility */
void lcd_putc( char znak )
{
lcd_data(znak);
}
/* Funkcia zobrazi na displeji nejaky text*/
void lcd_puts(char *string)
{
/* ToDo: toto je vasa uloha */
}
/* Funkcia nastavi kurzor na poziciu riadok, stlpec */
/* a text sa samozrejme vypise od kurzora dalej. */
void lcd_setCursor(char row, char col)
{
/* ToDo: aj toto je vasa uloha */
}
void lcd_clearline( unsigned char riadok )
{
unsigned char index;
lcd_setCursor( riadok, 0 );
for (index=1; index<20; index++) lcd_data( ' ' );
}
void lcd_clear( void )
{
lcd_clearline( 0 );
lcd_clearline( 1 );
lcd_clearline( 2 );
}
/* Funkcia zapne alebo vypne podsvietenie displeja */
void lcd_bklt( char OnOff)
{
if (OnOff)
DISPLAY_BKLT_high;
else
DISPLAY_BKLT_low;
}
/* Funkcia ulozi uzivatelom definovany znak do CG RAM na Address */
void def_znak(unsigned char *ZnakArray, unsigned char Address)
{
lcd_command(0x40|(Address<<3)); //nastavenie adresy znaku v CGRAM
for(unsigned char i = 0;i<8; i++) lcd_data( *(ZnakArray + i));
lcd_command(0x80); // zmena na DD RAM
}
#include <Arduino.h>
#include <SPI.h>
#include <dogm_7036.h>
/*Available functions in dogm_7036 Libraray:
void initialize (byte p_cs, byte p_si, byte p_clk, byte p_rs, byte p_res, boolean sup_5V, byte lines);
void string (const char *str);
void ascii (char character);
void position (byte column, byte line);
void displ_onoff (boolean on);
void cursor_onoff (boolean on);
void define_char (byte mem_adress, byte *dat);
void clear_display (void);
void contrast (byte contr);
*/
#define LED_D1 16
#define SW_A 17
#define SW_B 7
#define SW_C 8
// LCD Displej:
#define CLK 13 //(aka PB5)
#define SI 11 //(aka PB3)
#define RS 2 // (aka PD2)
#define CS 4 // (aka PD4)
#define BKLT 6 // (aka PD6)
dogm_7036 LCD;
int BL_pin = 6; // BL je na pine D6
void init_backlight();
void mono_backlight(byte brightness);
void setup()
{
init_backlight(); //use mono backlight in this sample code. Please change it to your configuration
// CS, RS
LCD.initialize(CS,SI,CLK,RS,4,1,DOGM163); //SS = 10, 0,0= use Hardware SPI, 9 = RS, 4 = RESET, 1 = 5V, EA DOGM163-A (=3 lines)
LCD.displ_onoff(true); //turn Display on
LCD.cursor_onoff(true); //turn Curosor blinking on
LCD.clear_display(); //Clear the whole content
LCD.position(1,1); //set Position: first line, first character
LCD.string("UAMT FEI STU"); //show String
LCD.position(1,2); //set Position: second line, first character
LCD.string("5. aprila 2024");
LCD.position(1,3);
LCD.string("3 lines 16 char");
mono_backlight(255); //full
delay(2000);
}
int con=0;
int mode=0;
int pod=0xff;
char buffer[16];
void loop()
{
/* sem dame zmeny kontrastu a podsvietenia cez tlacitka */
if ( digitalRead(SW_A)==0)
if (mode)
LCD.contrast(con--);
else
mono_backlight(pod--);
if ( digitalRead(SW_B)==0)
if (mode)
LCD.contrast(con++);
else
mono_backlight(pod++);
if (con<=0) con =1;
if (con>=63) con = 63;
if (pod<=0) pod = 1;
if (pod>=255) pod = 255;
if ( digitalRead(SW_C)==0)
{ digitalWrite(LED_D1,HIGH);
mode = !mode;
}
else
digitalWrite(LED_D1,LOW);
sprintf(buffer, "Podsviet: %02X ", pod);
LCD.position(1,2);
// 0123456789012345
LCD.string(buffer);
sprintf(buffer, "Contrast: %02d ", con);
LCD.position(1,3);
// 0123456789012345
LCD.string(buffer);
delay(100);
}
void init_backlight()
{
pinMode(BL_pin, OUTPUT);
mono_backlight(255);
}
void mono_backlight(byte brightness)
{
analogWrite(BL_pin, brightness);
}
Dokumentácia
- Datasheet displeja EA-DOGM163
- Datasheet radiča displeja ST7036 s tabuľkou inštrukcií a mapou znakov
- Stránka výrobcu displejov Display Visions
- Simulátor displeja pre Win
Inštrukcie radiča LCD displeja
Úloha
Upravte vzorový program tak, aby sa na zobrazovanej pozícii nezobrazoval kurzor. Požadovaný príkaz Cursor on/off (C) nájdete v tabuľke s inštrukciami.
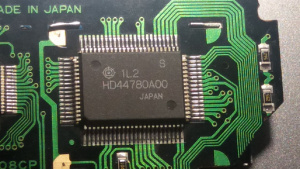
Okrem zobrazovania jednotlivých znakov dokážeme pomocou riadiacich príkazov lcd_command()
ovládať spôsob zobrazenia, meniť aktuálnu polohu kurzora, ako aj to či vôbec bude kurzor zobrazený, alebo nie. Príkladom jednej z najjednoduchších inštrukcií je 0000 0001
, ktorým sa vymaže celý zobrazený obsah a kurzor sa vráti do počiatočenej pozície 0,0 - pozri prvý riadok v tabuľke nižšie.
V jazyku C sa dané inštrukcie dajú posielať pomocou príkazu lcd_command()
, ktorý máte definovaný v lcd.h
Nasledovné inštrukcie ilustrujú využitie, pričom pre správne pochopenie by ste mali nahliadnuť aj do tabuľky nižšie.
lcd_command(0x01); /* Clear screen - vid riadok 1 v tabulke */
lcd_command(0x02); /* Cursor to home position - vid riadok 2 v tabulke */
Prípadne si to môžete zapísať aj prehľadnejšie pomocou už známych operácií s bitmi:
#define LCD_CLR 0 /* DB0: clear display */
#define LCD_HOME 1 /* DB1: return to home position */
lcd_command(1<<LCD_CLR); /* Clear screen */
lcd_command(1<<LCD_HOME); /* Set cursor to home position */
Iným užitočným a často používaným príkazom je nastavenie aktuálnej pozície kurzora, teda miesto na displeji, kam sa bude vypisovať text. Je to vlastne nastavenie adresy DDRAM, pričom jednotlivé adresy zodpovedajú pozíciam na displeji podľa tejto tabuľky.
// Nastavíme kurzor na 1. riadok pozicia 03 (4. v poradi) - na obr. pismeno E
lcd_command(0x80 + 3); // 0b1000 0000 + 3
// Nastavíme kurzor na 3. riadok pozicia 13 - na obr. pismeno t
lcd_command(0x80 + 0x20 + 13); // (0b1000 0000 + 0x2D)
Kompletná inštrukčná sada radiča HD44780 je uvedená v nasledovnej tabuľke:
Instruction | Code | Description | Execution time (max) (when fcp = 270 kHz) | |||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|
RS | R/W | D7 | D6 | D5 | D4 | D3 | D2 | D1 | D0 | |||
Clear display | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | Clears display and returns cursor to the home position (address 0). | 1.52 ms |
Cursor home | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | * | Returns cursor to home position. Also returns display being shifted to the original position. DDRAM content remains unchanged. | 1.52 ms |
Entry mode set | 0 | 0 | 0 | 0 | 0 | 0 | 0 | 1 | I/D | S | Sets cursor move direction (I/D); specifies to shift the display (S). These operations are performed during data read/write. | 37 μs |
Display on/off control | 0 | 0 | 0 | 0 | 0 | 0 | 1 | D | C | B | Sets on/off of all display (D), cursor on/off (C), and blink of cursor position character (B). | 37 μs |
Cursor/display shift | 0 | 0 | 0 | 0 | 0 | 1 | S/C | R/L | * | * | Sets cursor-move or display-shift (S/C), shift direction (R/L). DDRAM content remains unchanged. | 37 μs |
Function set | 0 | 0 | 0 | 0 | 1 | DL | N | F | * | * | Sets interface data length (DL), number of display line (N), and character font (F). | 37 μs |
Set CGRAM address | 0 | 0 | 0 | 1 | CGRAM address | Sets the CGRAM address. CGRAM data are sent and received after this setting. | 37 μs | |||||
Set DDRAM address | 0 | 0 | 1 | DDRAM address | Sets the DDRAM address. DDRAM data are sent and received after this setting. | 37 μs | ||||||
Read busy flag & address counter |
0 | 1 | BF | CGRAM/DDRAM address | Reads busy flag (BF) indicating internal operation being performed and reads CGRAM or DDRAM address counter contents (depending on previous instruction). | 0 μs | ||||||
Write CGRAM or DDRAM |
1 | 0 | Write Data | Write data to CGRAM or DDRAM. | 37 μs | |||||||
Read from CG/DDRAM | 1 | 1 | Read Data | Read data from CGRAM or DDRAM. | 37 μs | |||||||
Instruction bit names — I/D - 0 = decrement cursor position, 1 = increment cursor position; S - 0 = no display shift, 1 = display shift; D - 0 = display off, 1 = display on; C - 0 = cursor off, 1 = cursor on; B - 0 = cursor blink off, 1 = cursor blink on ; S/C - 0 = move cursor, 1 = shift display; R/L - 0 = shift left, 1 = shift right; DL - 0 = 4-bit interface, 1 = 8-bit interface; N - 0 = 1/8 or 1/11 duty (1 line), 1 = 1/16 duty (2 lines); F - 0 = 5×8 dots, 1 = 5×10 dots; BF - 0 = can accept instruction, 1 = internal operation in progress. |
Vysvetlivky:
CGRAM je pamäť tvarov špeciálnych znakov, ktoré si môže užívateľ nadefinovať sám (Character Generator RAM)
DDRAM je pamäť aktuálne zobrazených znakov (Display Data RAM). Každý zobrazený znak zodpovedá jednej pozícii v DDRAM. Prvý riadok má adresy od 0 po 1F pre 16 znakov. Druhý riadok má pridelené adresy 20 až 2F. Tretí riadok má potom adresy 30 až 3F.
Doba trvania (execution time) je stanovená pre oscilátor s frekvenciou 270 kHz. Avšak táto frekvencia záleží aj od napájacieho napätia a ďalších faktorov a môže sa pohybovať od 190 kHz po 350 kHz, takže ak nepoužívame bit BUSY ale pevné čakacie slučky, treba to zobrať do úvahy.
Výpis textových reťazcov
Úloha:
Namiesto vypisovania textov po jednom znaku si naprogramujte vlastnú funkciu na výpis textového reťazca. Potom demonštrujte jej využitie tak, že na tretí riadok displeja vypíšete svoje meno.
void lcd_puts(const char *s); /* deklaracia funkcie */
void lcd_puts(const char *s) /* definicia funkcie */
{
// sem pride vas vlastny kod...
}
void lcd_setCursor(char row, char col); /* deklaracia funkcie */
void lcd_setCursor(char row, char col) /* definicia funkcie */
{
// sem pride vas vlastny kod...
}
lcd_setCursor(3,3); // nastav kurzor na 3. riadok, 3. stlpec
lcd_puts("Meno Priezvisko");
Často však potrebujeme vypisovať aj hodnoty premenných a náročnejšie formátované výstupy. Ak vám chýba funkcia printf()
s jej rozsiahlymi formátovacími možnosťami, oceníte ukážku využitia pomocou jej sesterskej funkcie sprintf()
, ktorá vám pripraví požadovaný reťazec na výstup.
/* zadefinujeme pole znakov, t.j. reťazec dlhý ako je riadok na displeji
* t.j. 16 plus jeden naviac na ukončovací znak reťazca \0 */
// 01234567890123456 +1 na koniec retazca
char riadok[]= {" "};
int value = 42; // premenná, ktorej hodnotu by sme chceli zobraziť na LCD
sprintf(riadok,"Dec %d = %03X Hex",value,value); // Zobrazíme ju 2x: raz desiatkovo a potom hexa
lcd_puts(riadok);
Vytváranie vlastných znakov
Displej (resp. jeho radič HD44780) okrem iného obsahuje aj malú pamäť vyhradenú na užívateľom vytvorené znaky. Môže ich byť max. 8 a prístupné sú ako znaky s ASCII kódmi 0x00 až 0x08. S prvým zo znakov, ktorého kód je 0x00 je trocha problém, pretože aj reťazce v jazyku C používajú tento znak ako príznak konca reťazca. Znaky sa vytvárajú v matici 5x8 a ukladajú sa po riadkoch do pamäti CGRAM (Character Generator RAM) a mapujú sa na pozície 0-8 v DD RAM (Display Data RAM), alebo zrkadlene na adresy 9-F.
Vytváranie znakov ilustruje nasledovný obrázok. Keďže na hodnote najvyšších troch bitov nezáleží, budeme uvažovať s nulou.
Úloha:
Vypíšte na displej teplotu v stupňoch Celzia a svoje meno alebo iné slovo s diakritikou.
// vytvorenie specialneho znaku, ulozeny v IRAM, potom ho ulozime do CGRAM
unsigned char Znak_SC[8]= {0x1A,0x1D,0x04,0x04,0x04,0x05,0x02,0};// st.C
// zapis specialneho znaku do CGRAM na poziciu 4
def_znak( Znak_SC,4);// supen Celzia
lcd_data(0x04); // znak zadany priamo kodom
alebo ako súčasť reťazca
// 0123456789012345
lcd_puts("Teplota: 37\x04");
Zoznam úloh na odovzdanie
- Upravte vzorový program tak, aby sa na zobrazovanej pozícii nezobrazoval kurzor.
- Naprogramujte vlastnú funkciu na výpis textového reťazca. Demonštrujte jej využitie tak, že na druhý riadok displeja vypíšete svoje meno.
- Vypíšte na displej teplotu v stupňoch Celzia a svoje meno alebo iné slovo s diakritikou.
Zoznam bonusových úloh
2b. Pripojte k mikropočítaču dve tlačítka, pomocou ktorých budete text na displeji posúvať vľavo a vpravo.
5b. Zadefinujte vlastný špeciálny znak (znak s diakritikou) na pozíciu 0 (nula) CGRAM a zobrazte pomocou funkcie printf ako súčasť formátovaného príkazu do druhého riadku.