Processing 7-segment display
Zo stránky SensorWiki
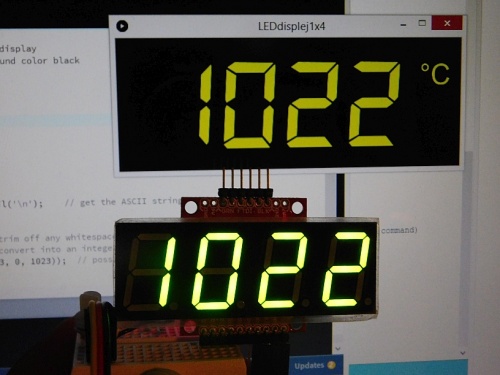
- Link to the necessary font: https://fontlibrary.org/en/font/segment7 - install before continuing.
Arduino code for the simple potentiometer measurement with the local displaying of the value on the OpenSegment display.
/*
Serial7Segment is an open source seven segment display.
Note: This code expects the display to be listening at 9600bps. If your display is not at 9600bps, you can
do a software or hardware reset. See the Wiki for more info:
http://github.com/sparkfun/Serial7SegmentDisplay/wiki/Special-Commands#wiki-baud
How to use the display:
//https://github.com/sparkfun/Serial7SegmentDisplay/wiki/Basic-Usage#wiki-cursor
To get this code to work, attached an Serial7Segment to an Arduino Uno using the following pins:
Pin 8 on Uno (software serial TX) to RX on Serial7Segment
VIN to PWR
GND to GND
*/
#include <SoftwareSerial.h>
SoftwareSerial LEDdisplej(2, 3); //RX pin, TX pin
int adcValue = 0;
void setup() {
Serial.begin(9600);
LEDdisplej.begin(9600); // Talk to the Serial7Segment at 9600 bps
LEDdisplej.write('v'); // Reset the display - this forces the cursor to return to the beginning of the display
}
void loop()
{
adcValue = 0; // Filter - average from 16 values
for (int i = 1; i<=16; i++)
adcValue = adcValue + analogRead(0);
adcValue = adcValue/16;
char tempString[10]; // Used for sprintf
sprintf(tempString, "%4d",adcValue); // Convert into a string that is right adjusted
//sprintf(tempString, "%d", adcValue); // Convert into a string that is left adjusted (requires digit 1 command)
//sprintf(tempString, "%04d", adcValue); // Convert into a string with leading zeros
//sprintf(tempString, "%4X", adcValue); // Convert int HEX, right adjusted
LEDdisplej.print(tempString); // Send serial string out the soft serial port to the S7S
Serial.println(tempString); // Works too, but we don't need trailing spaces ' ' in the front
// Serial.println(adcValue);
delay(100);
}
Corresponding Processing code for receiving the value from serial port and displaying it in the window same way as the OpenSegment did.
import processing.serial.*;
int adcValue = 0; // value received from Serial
// String Unit="mA";
// String Unit="kΩ";
// String Unit="°C"; // We can use Unicode chars directly
String Unit="\u00B0C"; // Unicode codes may be entered as well
Serial myPort;
PFont Segment, Units;
void setup() {
// Setup the display window
size(480, 180); // Size of the window
Segment = createFont("Segment7", 150); // Assign fonts and size
Units = createFont("Arial", 40);
textFont(Segment);
textAlign(RIGHT); // Text align
fill(250,250,0); // Font color is yellow = red + green
println(Serial.list()); // List all the available serial ports
// Then open the port you're using, my is the first, i.e. '0'
myPort = new Serial(this, Serial.list()[0], 9600);
// don't generate a serialEvent() unless you get a newline character:
myPort.bufferUntil('\n');
}
void draw()
{ // Let's start to display
background(0,0,0); // set the background color black
textFont(Segment);
text(adcValue, 400, 150);
textFont(Units);
text(Unit,465,65);
}
void serialEvent(Serial myPort)
{
String inString = myPort.readStringUntil('\n'); // get the ASCII string:
if (inString != null)
{
inString = trim(inString); // trim off any whitespace
adcValue = int(inString); // convert into an integer
adcValue =int(map(adcValue, 0, 1023, 0, 1023)); // possible range adjusting
}
}
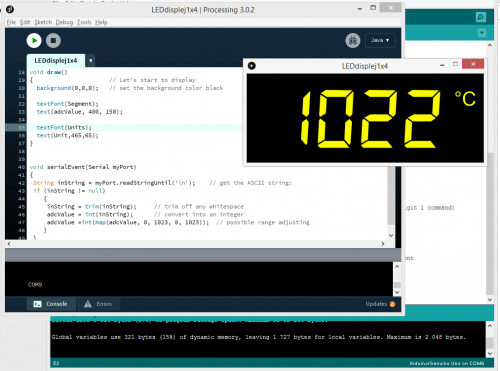